This problem is a slightly reworked version of a problem taken from hackerrank site. Follow the link for full description: https://www.hackerrank.com/challenges/python-sort-sort/problem. Find below a screenshot of the text:
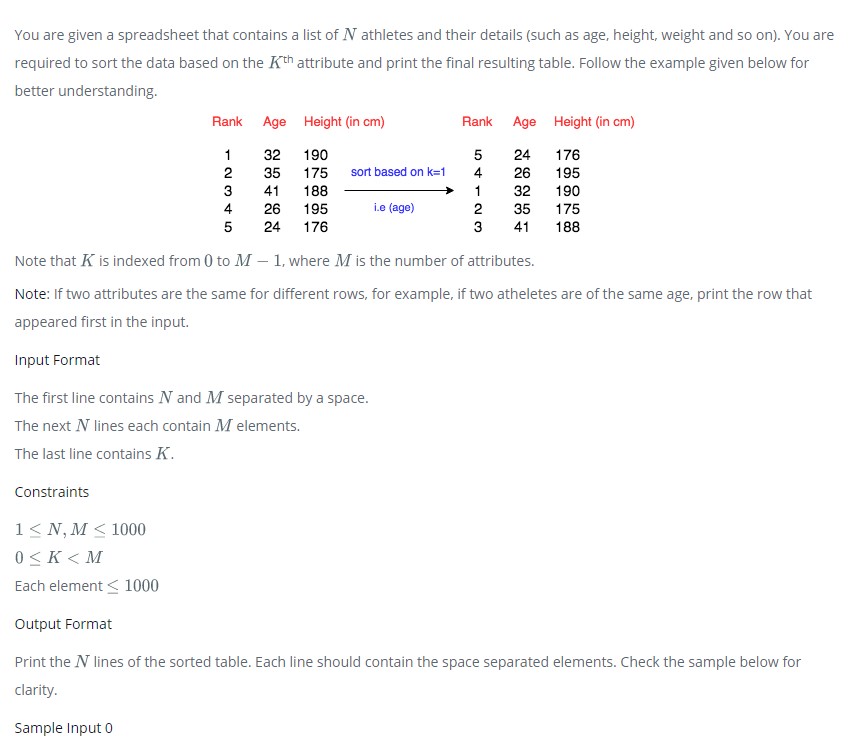
We select from the keyboard a series of numbers that build a database for a certain number of athletes. We decide the number of athletes we want to enter into our database, the number of attributes for each athlete, and the values of each attribute for each athlete. The problem requires to sort the database by the Kth attribute we give through the input() function. You can see my final code below.
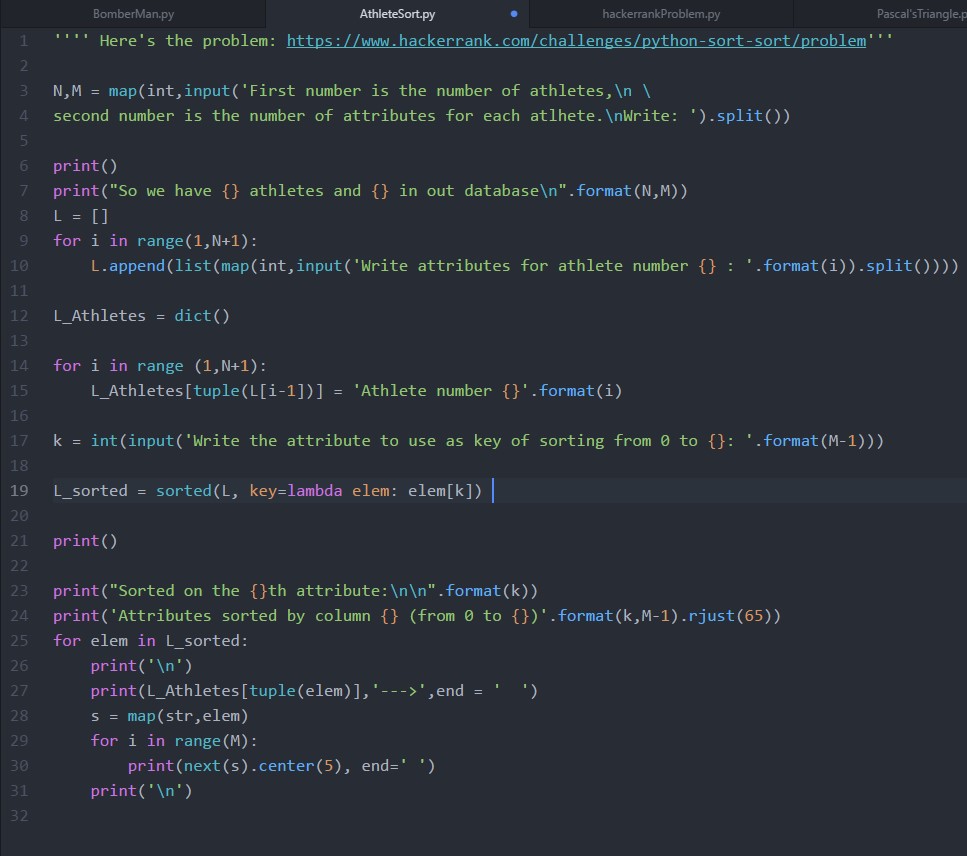
In lines 3-4 we take the numbers of athletes (N) and the number of attributes (M) through a
"combination" of the input() function, the split method and the map object.
In line 10 we build a list where each element is a list of the values of attributes for each athlete.
In line 14 we "hook" each athlete with his own attributes through a dictionary.
We change the type of the key from list to tuple because keys in a dictionary are immutable, therefore
a list cannot be a key in a dictionary because it's mutable.
In line 17 we take the Kth attribute from the keyboard (a number from 0 to M-1. We will not discuss at this stage
how to handle the error that happens if we give a value that is not included in this range).
Line 19 is important: we use the sorted method and the key optional parameter to sort the
list by the Kth index of each element of our list.
In line 25 we start a for loop in order to show the results in a nice design.
In line 28 we use the map object to convert each element of the element of the list into a string.
We need to proceed this way because the method center in line 30 is a string method, so it does not work with numbers.
We use the next function because the map object in Python 3 returns a generator, not a list.
The next function returns the next item from the iterator.
We shall now see the final output that we obtain when we give the same input written in the text of hackerrank site:
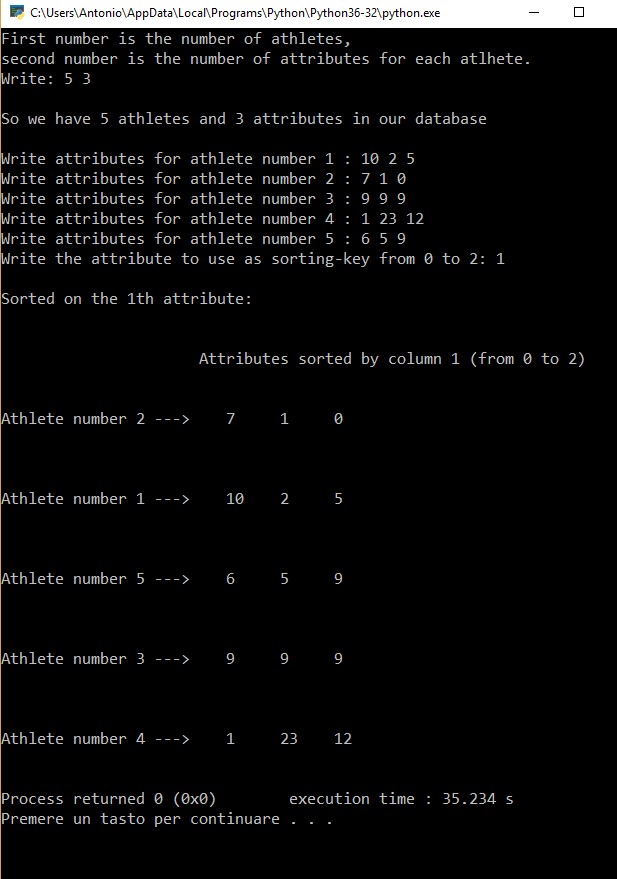
You can find my code here: GitHub Gist